In the process of Python Weibo crawling, proxy IP plays a vital role. It can help us break through many limitations and successfully obtain Weibo data. The following will introduce in detail the relevant techniques of using proxy IP in Python Weibo crawling.
1. Set proxy IP in Python
(I) Use requests library to set proxy IP
In Python's requests library, you can use proxy IP by setting the proxies parameter. For example, if we get an HTTP proxy IP of "123.45.67.89" and port of "8080", we can set it like this:
import requests
proxy = {
"http": "http://123.45.67.89:8080",
"https": "https://123.45.67.89:8080"
}
response = requests.get("https://weibo.com", proxies=proxy)
It should be noted here that if the proxy IP requires a username and password for verification, the username and password information must also be added to the proxy URL in the format of "http:// username: password @ proxy IP: port".
(II) Using proxy IP in Scrapy framework
If you use the Scrapy framework to crawl Weibo, it is also convenient to set the proxy IP. In Scrapy's settings.py file, you can set it as follows:
DOWNLOADER_MIDDLEWARES = {
'scrapy.downloadermiddlewares.httpproxy.HttpProxyMiddleware': 110,
'myproject.middlewares.ProxyMiddleware': 100,
}
PROXY_LIST = [
"http://123.45.67.89:8080",
"http://98.76.54.32:8888"
]
Then you need to create a middleware class called ProxyMiddleware, in which you can implement the logic of randomly selecting a proxy IP from PROXY_LIST and setting it to the request. The example is as follows:
import random
from scrapy.downloadermiddlewares.httpproxy import HttpProxyMiddleware
class ProxyMiddleware(HttpProxyMiddleware):
def \_\_init\_\_(self, proxy\_list):
self.proxy\_list = proxy\_list
@classmethod
def from\_crawler(cls, crawler):
return cls(
proxy\_list=crawler.settings.get('PROXY\_LIST')
)
def process\_request(self, request, spider):
proxy = random.choice(self.proxy\_list)
request.meta['proxy'] = proxy
With this setting, Scrapy will automatically use the randomly selected proxy IP when sending a request.
2. Management and maintenance of proxy IP
1. Construction and update of IP pool
In order to ensure the continuous crawling of Weibo, it is very necessary to build a proxy IP pool. The obtained multiple proxy IPs can be stored in a list or other data structure to form an IP pool. During the crawling process, when a proxy IP fails (such as being blocked or the connection timed out), the IP is removed from the IP pool and a new available proxy IP is added in time. New IP resources can be obtained from the proxy IP provider regularly to update the IP pool, for example, every 1-2 hours to ensure that there are enough available IPs in the IP pool.
(II) Error handling and IP switching
When using proxy IP to crawl Weibo data, it is inevitable to encounter various errors, such as connection rejection, timeout, etc. When these errors occur, reasonable error handling is required and the proxy IP is switched in time. The try-except block can be used in the code to capture exceptions during the request process. When an exception is captured, the currently used proxy IP is marked as unavailable, and a new proxy IP is selected from the IP pool to resend the request. For example:
while True:
try:
# Send a microblog crawling request using the current proxy IP
response = requests.get("https://weibo.com", proxies=proxy)
# If the request succeeds, process the response data
break
except requests.exceptions.RequestException as e:
# If the request fails, mark the current proxy IP as unavailable and switch the proxy IP
print(f"Request failed: {e}")
# Remove the current proxy IP from the IP pool
proxy\_pool.remove(proxy)
# Select a new proxy IP
if proxy\_pool:
proxy = random.choice(proxy\_pool)
else:
print("IP pool exhausted, waiting for new IP")
# You can add logic for adding new IPs here, such as pausing for a while and then reacquiring new IP resources
time.sleep(3600)
Through the above information about proxy IP By using various skills such as selection, setting, management and maintenance, we can better use proxy IP to break through restrictions in the process of Python Weibo crawling, improve the success rate and efficiency of crawling, and successfully obtain the required Weibo data, laying a solid foundation for subsequent data analysis, public opinion monitoring and other work. But at the same time, we must also pay attention to the relevant laws and regulations and the provisions of the Weibo platform when crawling Weibo, and carry out data crawling activities legally and compliantly.
Related Recommendations
- How to achieve efficient management of TikTok's multi-store operations?
- Shopify store operations: proxy IP ensures smooth access and data security
- How important is free testing of overseas agent IP?
- The important role of proxy IP in data collection and analysis
- How to evaluate the quality and actual effectiveness of overseas HTTP?
- Application of dynamic IP in preventing DDoS attacks
- How to choose a good foreign IP agent?
- Solve problems such as foreign trade companies 'overseas networks being stuck and unable to open
- The crawler encounters a Google Captcha proxy pool
- Can't you enter your favorite website? Here are efficient solutions!
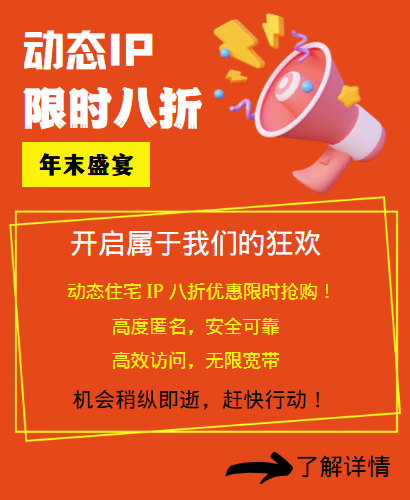