In crawler development, the use of proxy IP is essential to bypass the anti-crawler mechanism of the target website. However, in the process of collecting proxy IPs, duplicate IP addresses are often encountered. These duplicate IPs not only waste the resources of the crawler, but also may reduce the collection efficiency. Therefore, effectively removing duplicate proxy IP addresses is an important part of crawler development. This article will introduce several practical methods to remove duplicate proxy IPs in detail, and illustrate them with specific examples and codes.
1. Use Python Set to remove duplicates
A set in Python is an unordered data structure that does not contain duplicate elements. Using this feature, we can easily achieve the deduplication of proxy IPs.
1.1 Method Description
Convert the proxy IP list to a set, the set will automatically remove duplicate elements, and then convert the set back to a list.
1.2 Example code
# Assume we have a list containing duplicate proxy IPs
proxy_list = [
"192.168.1.1:8080",
"192.168.1.2:8080",
"192.168.1.1:8080", # Duplicate IP
"10.0.0.1:3128",
"172.16.0.1:80"
]
# Use sets to remove duplicates
unique_proxies = list(set(proxy_list))
# Print the proxy IP list after deduplication
print(unique_proxies)
1.3 Notes
- Since sets are unordered, the order of the list after deduplication may change.
- If the proxy IP list is very large, using sets may take up more memory.
2. Use Pandas library to remove duplicates
Pandas is a powerful Python data analysis library that provides a DataFrame data structure that can easily process tabular data. We can use Pandas's drop_duplicates
method to remove duplicate proxy IPs.
2.1 Method description
Convert the proxy IP list to a Pandas DataFrame, then use the drop_duplicates
method to remove duplicate rows, and finally convert the DataFrame back to a list.
2.2 Example code
import pandas as pd
# Assume we have a list containing duplicate proxy IPs
proxy_list = [
"192.168.1.1:8080",
"192.168.1.2:8080",
"192.168.1.1:8080", # Duplicate IP
"10.0.0.1:3128",
"172.16.0.1:80"
]
# Convert the list to a Pandas DataFrame
df = pd.DataFrame(proxy_list, columns=['Proxy'])
# Remove duplicates using the drop_duplicates method
df_unique = df.drop_duplicates()
# Convert the deduplicated DataFrame back to a list
unique_proxies = df_unique['Proxy'].tolist()
# Print the proxy IP list after deduplication
print(unique_proxies)
2.3 Notes
- Pandas library needs to be installed in advance, which can be installed through
pip install pandas
. - Pandas library has better performance when processing big data, but the amount of code is slightly more than that of collections.
3. Deduplication using hash table (Dictionary)
Hash table is a data structure based on key-value pairs. By using the unique key feature of hash table, we can also achieve deduplication of proxy IP.
3.1 Method description
Traverse the proxy IP list and store each IP as a key in the hash table. Since the key of the hash table is unique, duplicate IPs will be automatically ignored. Finally, convert the key of the hash table into a list.
3.2 Example code
# Assume we have a list containing duplicate proxy IPs
proxy_list = [
"192.168.1.1:8080",
"192.168.1.2:8080",
"192.168.1.1:8080", # Duplicate IP
"10.0.0.1:3128",
"172.16.0.1:80"
]
# Use hash table to remove duplicates
proxy_dict = {}
for proxy in proxy_list:
proxy_dict[proxy] = None
# Convert hash table keys to a list
unique_proxies = list(proxy_dict.keys())
# Print the proxy IP list after deduplication
print(unique_proxies)
3.3 Notes
- Hash tables are usually implemented in Python through dictionaries.
- Compared with sets and Pandas, the hash table deduplication method has a slightly larger amount of code, but has better performance when processing big data.
4. Summary
In crawler development, removing duplicate proxy IP addresses is an important step to ensure collection efficiency and quality. This article introduces three practical deduplication methods: using Python sets, Pandas libraries, and hash tables. Each method has its own unique advantages and applicable scenarios, and developers can choose the appropriate method according to actual needs. At the same time, in order to further improve the stability and efficiency of the crawler, you can also consider combining multiple deduplication methods, as well as regularly updating and maintaining the proxy IP list.
Related Recommendations
- U.S. Residential IP: Virtual Reality, a powerful tool for accessing overseas
- Why is transparency restricted? Can anonymity hide IP?
- Google Play Account Guide: The latest strategies for 2024
- What is the role of dynamic proxy IP in the e-commerce industry?
- In the development of the global Internet, what role does overseas dynamic and long-term ISPs play?
- Unlock proxy features in Windows: Enhance your privacy and online access
- Why is exclusive proxy IP more efficient in use?
- What are the advantages of exclusive IP?
- Enhance business operations: Use agents to improve performance
- Rotating agents play a key role in the advertising verification business
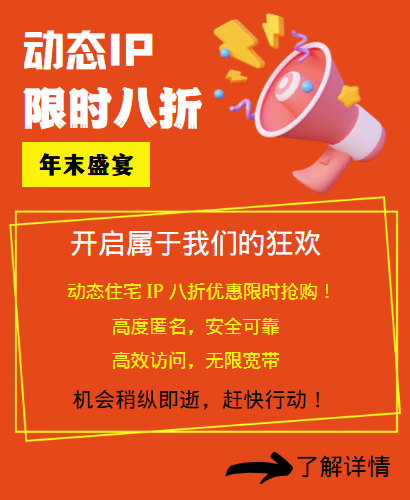