In network communications, proxy servers play an important role. They can hide the real IP address of the client and provide anonymity. However, the anonymity levels of proxy servers vary, and understanding these levels is essential to ensure network security and data integrity. This article will describe how to use PHP to detect the anonymity level of the proxy.
1. Classification of anonymity levels of proxy servers
The anonymity levels of proxy servers are generally divided into the following categories:
- Transparent Proxy:
- Forward the real IP address of the client.
- The server can easily identify the real IP of the client.
- Anonymous Proxy:
- Hide the real IP address of the client, but will reveal that a proxy is used.
- The server knows that the request is made through the proxy, but does not know the real IP of the client.
- Elite Proxy or High Anonymity Proxy:
- Completely hide all information of the client and only display the information of the proxy server.
- The server cannot recognize that the request is sent through a proxy, nor can it obtain the client's real IP.
2. Use PHP to detect the use of proxy and anonymity level
2.1 Detect whether a proxy is used
The $_SERVER
array in PHP contains a lot of information about client requests. By checking specific HTTP header fields, you can determine whether the client is using a proxy.
function isProxyUsed() {
// Check common proxy header fields
$proxyHeaders = array(
'HTTP_VIA',
'HTTP_X_FORWARDED_FOR',
'HTTP_X_REAL_IP',
'HTTP_CLIENT_IP',
'HTTP_FORWARDED'
);
foreach ($proxyHeaders as $header) {
if (!empty($_SERVER[$header])) {
return true; // Proxy is used
}
}
return false; // Proxy is not used
}
2.2 Get the real IP address of the client
When not using a proxy, you can directly get the real IP address of the client through $_SERVER['REMOTE_ADDR']
. But when using a proxy, additional processing is required.
function getClientRealIp() {
if (!empty($_SERVER['HTTP_CLIENT_IP'])) {
$ip = $_SERVER['HTTP_CLIENT_IP'];
} elseif (!empty($_SERVER['HTTP_X_FORWARDED_FOR'])) {
// Note: HTTP_X_FORWARDED_FOR may contain multiple IP addresses and need to be parsed
$ip = explode(',', $_SERVER['HTTP_X_FORWARDED_FOR'])[0];
} else {
$ip = $_SERVER['REMOTE_ADDR'];
}
return $ip;
}
2.3 Detecting the anonymity level of a proxy
To detect the anonymity level of a proxy, we can write a function that will check for specific header fields in the $_SERVER
array and determine the anonymity level of the proxy based on the values of those fields.
function detectProxyAnonymity() {
$realIp = getClientRealIp();
$serverIp = $_SERVER['REMOTE_ADDR'];
if ($realIp === $serverIp) {
// No proxy or transparent proxy
if (isProxyUsed()) {
return 'Transparent';
} else {
return 'No Proxy';
}
} else {
// Proxy is used, but not transparent proxy
$proxyHeaders = array(
'HTTP_VIA',
'HTTP_X_FORWARDED_FOR',
'HTTP_X_REAL_IP',
'HTTP_CLIENT_IP',
'HTTP_FORWARDED'
);
foreach ($proxyHeaders as $header) {
if (!empty($_SERVER[$header]) && strpos($_SERVER[$header], $realIp) !== false) {
// The proxy header field contains the client's real IP, but this is not a high anonymous proxy
return 'Anonymous';
}
}
// If no proxy header field contains the client's real IP, it is a high anonymous proxy
return 'Elite';
}
}
2.4 Example call
Here is a sample code on how to call the above function to detect the anonymity level of the proxy:
if (isProxyUsed()) {
$anonymityLevel = detectProxyAnonymity();
echo "Proxy Anonymity Level: " . $anonymityLevel;
} else {
echo "No proxy is being used.";
}
3. Notes
- Reliability of HTTP header fields:
- Not all proxies will set the above HTTP header fields.
- Some proxies may forge these fields, so the detection results may be inaccurate.
- Security:
- Relying on HTTP header fields to determine the anonymity level of a proxy may not be secure enough.
- In scenarios where high security is required, more reliable methods should be used to detect proxies.
- Performance:
- Frequently checking HTTP header fields may have an impact on performance.
- These checks should be used with caution in performance-sensitive applications.
With this article, you should be able to understand how to use PHP to detect the use of proxies and anonymity levels. However, please note that these methods are not absolutely reliable and may be affected by proxy configuration and behavior. In scenarios where high security is required, more reliable methods should be used to detect proxies.
Related Recommendations
- API proxy, a bridge connecting enterprises and markets
- How to evaluate cross-border e-commerce sellers 'stores?
- What is IP? How does IP work?
- Native static residential IP dual ISPs greatly improve network stability and reliability!
- IP address blocked? Practical tips for quickly replacing IP
- Infinite possibilities for overseas static residential IP agents
- Socks5 Agents: Strategies to Improve Online Privacy Protection
- Configuration and use of Amazon forward proxy
- What issues should I pay attention to in the application of computer room agent network?
- How to change the IP address?
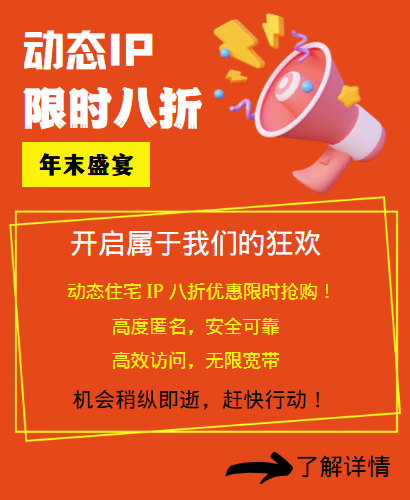