In application scenarios such as web crawlers, data collection, and SEO optimization, the proxy IP pool is a very important infrastructure. It can help you bypass the access restrictions of the target website, improve the success rate of data crawling, and protect your real IP address from being exposed. When you have obtained a large number of IP addresses, how to effectively build and manage a proxy IP pool has become an issue that needs to be explored in depth. This article will introduce in detail how to start from scratch and gradually build an efficient and reliable proxy IP pool.
1. Screening and verification of IP addresses
1.1 Preliminary screening
First, you need to perform a preliminary screening of the obtained IP addresses. This includes removing duplicate IPs, invalid IPs (such as private addresses, broadcast addresses, etc.), and those IPs that are obviously not in the public network range. This step can be done by writing simple scripts or using existing tools.
1.2 Verify validity
Next, you need to verify the validity of these IP addresses. This usually involves checking whether the IP is reachable, whether the port is open, and whether the proxy connection can be successfully established. You can use the ping command, telnet tool, or write a custom verification script to complete this step.
Sample code(Python):
import socket
def check_ip(ip, port):
try:
# Try to connect to IP and port
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.settimeout(1) # Set timeout to 1 second
s.connect((ip, port))
s.close()
return True
except Exception as e:
return False
# Sample IP list
ip_list = ['192.168.1.1', '8.8.8.8', '10.0.0.1'] # Please replace with the actual IP list
port = 8080 # Proxy port, adjust according to actual situation
# Verify IP validity
valid_ips = [ip for ip in ip_list if check_ip(ip, port)]
print("Valid IPs:", valid_ips)
2. Proxy IP Pool Construction
2.1 Database Design
In order to efficiently manage and schedule proxy IPs, you need to design a database to store relevant information about IP addresses. This information includes but is not limited to: IP address, port, status (available/unavailable), response time, last verification time, etc.
2.2 Database Construction
You can choose to use relational databases such as MySQL and PostgreSQL, or NoSQL databases such as MongoDB and Redis. Here, taking MySQL as an example, you can create a database named proxy_pool
and create a table named proxies
in it to store proxy IP information.
Sample SQL statement:
CREATE DATABASE proxy_pool;
USE proxy_pool;
CREATE TABLE proxies (
id INT AUTO_INCREMENT PRIMARY KEY,
ip VARCHAR(15) NOT NULL,
port INT NOT NULL,
status ENUM('available', 'unavailable') DEFAULT 'unavailable',
response_time FLOAT DEFAULT NULL,
last_checked TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
2.3 Implement scheduling logic
Next, you need to write a scheduler to manage the allocation and recycling of proxy IPs. This scheduler should be able to intelligently select the optimal proxy IP for allocation based on information such as IP status and response time. At the same time, it also needs to regularly verify the validity of the proxy IP and update the status information in the database.
Sample code (Python, using SQLAlchemy and thread pool):
from sqlalchemy import create_engine, Column, Integer, String, Enum, Float, DateTime
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
from concurrent.futures import ThreadPoolExecutor
import time
# Database configuration
DATABASE_URI = 'mysql+pymysql://username:password@localhost/proxy_pool'
# Create database engine and session
engine = create_engine(DATABASE_URI)
Base = declarative_base()
Session = sessionmaker(bind=engine)
session = Session()
# Define proxy IP model
class Proxy(Base):
__tablename__ = 'proxies'
id = Column(Integer, primary_key=True)
ip = Column(String(15), nullable=False)
port = Column(Integer, nullable=False)
status = Column(Enum('available', 'unavailable'), default='unavailable')
response_time = Column(Float, default=None)
last_checked = Column(DateTime, default=time.strftime('%Y-%m-%d %H:%M:%S'))
# Initialize the database
Base.metadata.create_all(engine)
# Function to verify the proxy IP
def check_proxy(proxy):
# The actual verification logic is omitted here, just as an example
# You can write verification code according to actual needs
proxy.status = 'available' # Assume that the verification is successful
proxy.response_time = 0.1 # Assume that the response time is 0.1 seconds
proxy.last_checked = time.strftime('%Y-%m-%d %H:%M:%S')
session.add(proxy)
session.commit()
# Scheduler
def schedule_proxies():
while True:
proxies = session.query(Proxy).filter(Proxy.status == 'unavailable').all()
with ThreadPoolExecutor(max_workers=10) as executor:
futures = [executor.submit(check_proxy, proxy) for proxy in proxies]
for future in futures:
future.result() # Wait for all tasks to complete
time.sleep(60) # Check every 60 seconds
# Start the scheduler
if __name__ == '__main__':
schedule_proxies()
3. Optimization and maintenance of proxy IP pool
3.1 Load balancing
To balance the load of proxy IP, you can implement a simple load balancing algorithm, such as round robin. Robin), random selection or weighted random selection, etc. In this way, each proxy IP can be used relatively evenly, avoiding being blocked or performance degradation due to excessive use of a certain IP.
3.2 Failure retry
In actual applications, proxy IPs may fail for various reasons (such as the target website updating the anti-crawler strategy, proxy server failure, etc.). Therefore, you need to implement a failure retry mechanism, which can automatically try to use other available proxy IPs for retry when a proxy IP fails.
3.3 Scheduled cleanup
Over time, some proxy IPs may become unavailable due to long-term non-use or verification failure. Therefore, you need to regularly clean up these invalid proxy IPs to keep the proxy IP pool clean and efficient. You can set a scheduled task to clean up invalid proxy IPs every once in a while.
3.4 Monitoring and alarm
In order to promptly discover and solve problems in the proxy IP pool, you need to implement a monitoring and alarm system. This system can monitor the usage, response time, error rate and other indicators of proxy IP, and issue alarm information in time when an abnormality occurs (such as sending emails, SMS or triggering Webhook, etc.).
Conclusion
Building an efficient and reliable proxy IP pool requires comprehensive consideration of multiple aspects, including the screening and verification of IP addresses, the design and management of databases, the implementation and optimization of scheduling logic, etc. Through the introduction and sample code of this article, I believe you have a preliminary understanding and knowledge of how to build a proxy IP pool. Of course, this is just a starting point, and you can also make more customization and optimization according to actual needs. I hope this article can help you!
Related Recommendations
- Detailed explanation of tunnel agent technology: principles, applications and advantages
- The role of U.S. residential agent IP in Amazon's North American site operations
- What factors should be considered when selecting a residential agent IP?
- Why does SEO optimization require proxy IP
- Tailor Your Internet Access with 98IP Home Residential IPs
- 98IP tells you the key points of distributed crawler design
- Benefits of using iPhone proxy servers to enhance online security and privacy
- How to log in to social accounts in batches: Proxy IP switching steps and considerations
- Exclusive static residential IP: stable, high-speed, and secure, creating a dedicated network channel
- How effective is the exclusive IP dedicated to FaceBook? How to get it?
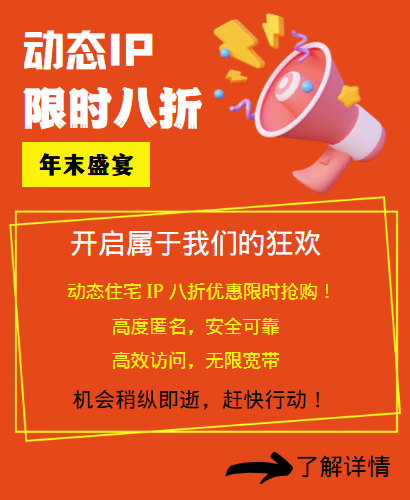